Block Bad Signups with the Real-Time Email Validation API
An unprotected signup form leaves your business vulnerable to fake or even harmful data entries that jeopardize your security. With a real-time email validation API, ZeroBounce automatically detects invalid and high-risk data and blocks it from ever entering your email and user database.
Verify Email Addresses on your Web Forms in Real-Time
Name
John Doe
Password
**********
API Results
Fast, secure email API service

99% accuracy guaranteed
No other email checker API offers our accuracy and precision. Spot over 30 email types in bulk or real time with a money-back guarantee.

Real-time results
ZeroBounce handles email validation in real time. As users enter their email addresses on your registration forms, we detect the email type and block or allow it accordingly.

Rapid email verification
Our real-time verification API is almost instant. Validate a single email address in just 3 seconds. Clean your entire list in under 1 hour.

Advanced API security
Privacy and security are the priority. We use military-grade data encryption and continuously improve our email list cleaningⓘThe process of removing invalid and high-risk emails, such as spam traps or disposable emails, from an email list. Email list cleaning can be performed after gathering data via email validation. API validation schema to filter out bad requests.
The No. 1 email validationⓘA process that determines if an email address uses valid syntax, exists on a given domain, and is configured to receive incoming email messages service for 400,000+ clients
Why choose the ZeroBounce email validator API?
Implementing our email hygiene API gives your company the extra defense it needs against invalid and high-risk emails.

Real-time email verification
Automatically detect low-quality emails at the point of registration on your website or landing page. Block invalid, disposable emailsⓘA temporary email address that users can create using a temporary email website or creation tool. You can use a disposable email address for a brief period before it expires and becomes invalid., spam traps, and more. With ZeroBounce, risky emails don’t stand a chance.

Fewer email bounces
When you keep invalid emails off your list, you can be confident that your emails will hit the inbox. The email validation API not only cleans your existing database but safeguards you against unnecessary email bounces 24/7.

Better email marketing ROI
An API email checking service is mandatory for the modern email marketer. By proactively cleaning your emails, more of your emails reach the inbox. That means more opens, clicks, and eyes on your content.
How our email API service works
Real-time email verification API
Validate emails instantly
Easy to install and great at keeping your data fresh! Prevent typos, known complainers, fake and poor-quality email addresses from getting on your lists. Plus, get full usage reports and insights into your email hygiene.
Complete software development kit (.NET, PHP, Python, Java, Swift and more)
Integrates into your favorite platforms
Geo-redundant servers for availability
$response = ZeroBounce::Instance()->validate(
"<EMAIL_ADDRESS>", // The email address you want to validate
"<IP_ADDRESS>" // The IP Address the email signed up from (Can be blank)
);
// can be: valid, invalid, catch-all, unknown, spamtrap, abuse, do_not_mail
$status = $response->status;
let email = "<EMAIL_ADDRESS>" // The email address you want to validate
let ipAddress = "127.0.0.1" // The IP Address the email signed up from (Optional)
ZeroBounceSDK.shared.validate(email: email, ipAddress: ipAddress) { result in
switch result {
case .Success(let response):
NSLog("validate success response=\(response)")
case .Failure(let error):
NSLog("validate failure error=\(String(describing: error))")
switch error as? ZBError {
case ZBError.notInitialized:
break
case ZBError.decodeError(let messages):
/// decodeError is used to extract and decode errors and messages
/// when they are not part of the response object
break
default:
break
}
}
}
ZeroBounceSDK.getInstance().validate(
"<ANY_EMAIL_ADDRESS>",
"<OPTIONAL_IP_ADDRESS>",
new ZeroBounceSDK.OnSuccessCallback<ZBValidateResponse>() {
@Override
public void onSuccess(ZBValidateResponse response) {
System.out.println("validate response=" + response.toString());
}
}, new ZeroBounceSDK.OnErrorCallback() {
@Override
public void onError(String errorMessage) {
System.out.println("validate error=" + errorMessage);
}
});
);
let activity_data = ZeroBounce::new(ZERO_BOUNCE_API_KEY)
.validate_email("valid@example.com")?;
println!("Activity data: {:#?}", activity_data);
Zerobounce.validate('valid@example.com')
# Zerobounce.validate('valid@example.com', '192.168.0.1') # optional IP address
=>
{"address"=>"valid@example.com",
"status"=>"valid",
"sub_status"=>"",
"free_email"=>false,
"did_you_mean"=>nil,
"account"=>nil,
"domain"=>nil,
"domain_age_days"=>"9692",
"smtp_provider"=>"example",
"mx_found"=>"true",
"mx_record"=>"mx.example.com",
"firstname"=>"zero",
"lastname"=>"bounce",
"gender"=>"male",
"country"=>nil,
"region"=>nil,
"city"=>nil,
"zipcode"=>nil,
"processed_at"=>"2023-04-28 15:18:45.631"}
from zerobouncesdk import ZeroBounce, ZBException
zero_bounce = ZeroBounce("<YOUR_API_KEY>")
email = "<EMAIL_ADDRESS>" // The email address you want to validate
ip_address = "127.0.0.1" // The IP Address the email signed up from (Optional)
try:
response = zero_bounce.validate(email, ip_address)
print("ZeroBounce validate response: " + response)
except ZBException as e:
print("ZeroBounce validate error: " + str(e))
try
ZBSetApiKey('YOUR__API__KEY');
response := ZbValidateEmail('possible_trap@example.com', '99.110.204.1');
WriteLn('Validation status: ', response.Status);
WriteLn('Validation sub status: ', response.SubStatus);
except on e: Exception do
begin
WriteLn('Exception occured:');
WriteLn(e.Message);
end;
end;
try
ZBSetApiKey('YOUR__API__KEY');
response := ZbValidateEmail('possible_trap@example.com', '99.110.204.1');
WriteLn('Validation status: ', response.Status);
WriteLn('Validation sub status: ', response.SubStatus);
except on e: Exception do
begin
WriteLn('Exception occured:');
WriteLn(e.Message);
end;
end;
ZeroBounceSDK.getInstance().validate(
"<ANY_EMAIL_ADDRESS>",
"<OPTIONAL_IP_ADDRESS>",
new ZeroBounceSDK.OnSuccessCallback<ZBValidateResponse>() {
@Override
public void onSuccess(ZBValidateResponse response) {
System.out.println("validate response=" + response.toString());
}
}, new ZeroBounceSDK.OnErrorCallback() {
@Override
public void onError(String errorMessage) {
System.out.println("validate error=" + errorMessage);
}
});
);
const email = "<EMAIL_ADDRESS>"; // The email address you want to validate
const ip_address = "127.0.0.1"; // The IP Address the email signed up from (Optional)
try {
const response = await zeroBounce.validateEmail(email, ip_address);
} catch (error) {
console.error(error);
}
const email = "<EMAIL_ADDRESS>"; // The email address you want to validate
const ip_address = "127.0.0.1"; // The IP Address the email signed up from (Optional)
try {
const response = await zeroBounce.validateEmail(email, ip_address);
} catch (error) {
console.error(error);
}
var email = "<EMAIL_ADDRESS>"; // The email address you want to validate
var ipAddress = "127.0.0.1"; // The IP Address the email signed up from (Optional)
ZeroBounce.Instance.Validate(email, ipAddress,
response =>
{
Debug.WriteLine("Validate success response " + response);
// ... your implementation
},
error =>
{
Debug.WriteLine("Validate failure error " + error);
// ... your implementation
});
ZeroBounceSDK.validate(
"<EMAIL_TO_TEST>",
"<OPTIONAL_IP_ADDRESS>",
{ rsp ->
Log.d("MainActivity", "validate rsp: $rsp")
// your implementation
},
{ error ->
Log.e("MainActivity", "validate error: $error")
// your implementation
}
)
zerobouncego.APIKey = "... Your API KEY ..."
// For Querying a single E-Mail and IP
// IP can also be an empty string
response, error_ := zerobouncego.Validate("possible_typo@example.com", "123.123.123.123")
if error_ != nil {
fmt.Println("error occurred: ", error_.Error())
} else {
// Now you can check status
if response.Status == zerobouncego.S_INVALID {
fmt.Println("This email is valid")
}
// .. or Sub-status
if response.SubStatus == zerobouncego.SS_POSSIBLE_TYPO {
fmt.Println("This email might have a typo")
}
}
// #include <ZeroBounce/ZeroBounce.h>
ZeroBounce* zb = zero_bounce_get_instance();
zero_bounce_initialize(zb, "<YOUR_API_KEY>");
void on_error(ZBErrorResponse error_response) {
printf("%s
", zb_error_response_to_string(&error_response));
}
void on_success_validate(ZBValidateResponse response) {
printf("%s
", zb_validate_response_to_string(&response));
}
char* email = "valid@example.com"; // The email address you want to validate
char* ip_address = "127.0.0.1"; // The IP Address the email signed up from (Optional)
validate_email(zb, email, ip_address, on_success_validate, on_error);
// #include <ZeroBounce/ZeroBounce.h>
ZeroBounce::getInstance()->initialize("<YOUR_API_KEY>");
std::string email = "valid@example.com";
std::string ipAddress = "127.0.0.1";
ZeroBounce::getInstance()->validate(
email,
ipAddress,
[](ZBValidateResponse response) {
cout << response.toString() << endl;
},
[](ZBErrorResponse errorResponse) {
cout << errorResponse.toString() << endl;
}
);
Bulk email verificationⓘThe process of determining if multiple email addresses are real and configured to receive incoming email messages. API
Bulk email verifier script
The bulk email verifier API is ideal for those who frequently want to clean their email list. Automate the process, save time, and let the API handle it. Call the API with one of 16 programming languages, including a .NET, C#, Javascript, and PHP bulk email verifier script.
Complete software development kit (.NET, PHP, Python, Java, Swift and more)
Integrates into your favorite platforms
Geo-redundant servers for availability
$response = ZeroBounce::Instance()->sendFile(
"<FILE_PATH>", // The csv or txt file
"<EMAIL_ADDRESS_COLUMN>", // The column index of the email address in the file. Index starts at 1
"<RETURN_URL>", // The URL will be used as a callback after the file is sent
"<FIRST_NAME_COLUMN>", // The column index of the user's first name in the file
"<LAST_NAME_COLUMN>", // The column index of the user's last name in the file
"<GENDER_COLUMN>", // The column index of the user's gender in the file
"<IP_ADDRESS_COLUMN>", // The column index of the IP address in the file
"<HAS_HEADER_ROW>" // If the first row from the submitted file is a header row. True or False
);
$fileId = $response->fileId; // e.g. "aaaaaaaa-zzzz-xxxx-yyyy-5003727fffff"
let filePath = File("<FILE_PATH>") // The csv or txt file
let emailAddressColumn = 3 // The index of "email" column in the file. Index starts at 1
let firstNameColumn = 3 // The index of "first name" column in the file
let lastNameColumn = 3 // The index of "last name" column in the file
let genderColumn = 3 // The index of "gender" column in the file
let ipAddressColumn = 3 // The index of "IP address" column in the file
let hasHeaderRow = true // If this is 'true' the first row is considered as table headers
let returnUrl = "https://domain.com/called/after/processing/request"
ZeroBounceSDK.shared.sendFile(
filePath: filePath,
emailAddressColumn: emailAddressColumn,
returnUrl: returnUrl,
firstNameColumn: firstNameColumn,
lastNameColumn: lastNameColumn,
genderColumn: genderColumn,
ipAddressColumn: ipAddressColumn,
hasHeaderRow: hasHeaderRow
) { result in
switch result {
case .Success(let response):
NSLog("sendFile success response=\(response)")
case .Failure(let error):
NSLog("sendFile failure error=\(String(describing: error))")
}
}
File myFile = new File("<FILE_PATH>"); // The csv or txt file
int emailAddressColumn = 3; // The column index of the email address in the file. Index starts at 1
ZeroBounceSDK.getInstance().sendFile(
myFile,
emailAddressColumn,
null,
new ZeroBounceSDK.OnSuccessCallback<ZBSendFileResponse>() {
@Override
public void onSuccess(ZBSendFileResponse response) {
System.out.println("sendFile response=" + response.toString());
}
}, new ZeroBounceSDK.OnErrorCallback() {
@Override
public void onError(String errorMessage) {
System.out.println("sendFile error=" + errorMessage);
}
});
let file_content = String::from("")
+ "invalid@example.com
"
+ "valid@example.com
"
+ "toxic@example.com
"
+ "donotmail@example.com
";
// initialize ZBFile to be used for bulk request
let file_content_vec = Vec::from(file_content);
let zb_instance = ZeroBounce::new(ZERO_BOUNCE_API_KEY);
let mut zb_file = ZBFile::from_content(file_content_vec);
// Alternatively:
// let mut zb_file = ZBFile::from_path("/path/to/file.csv".to_string());
// customize file
zb_file = zb_file
.set_remove_duplicate(false)
.set_has_header_row(false);
// submit file for bulk validation
let submit_inst = zb_instance.bulk_validation_file_submit(&zb_file)?;
// extract file_id (to use in future endpoints)
let file_id_string = submit_inst.file_id.unwrap();"
validate_file_path = File.join(Dir.pwd, 'files', 'validation.csv')
=> "/home/zb/wrappers/ruby-test/files/validation.csv"
Zerobounce.validate_file_send(validate_file_path)
=>
{"success"=>true,
"message"=>"File Accepted",
"file_name"=>"validation.csv",
"file_id"=>"75d854a6-565c-49f9-b4c8-b3344480ec4c"}
from zerobouncesdk import ZeroBounce, ZBException
zero_bounce = ZeroBounce("<YOUR_API_KEY>")
file_path = './email_file.csv' // The csv or txt file
email_address_column = 1 // The index of "email" column in the file. Index starts at 1
return_url = "https://domain.com/called/after/processing/request"
first_name_column = None // The index of "first name" column in the file
last_name_column = None // The index of "last name" column in the file
gender_column = None // The index of "gender" column in the file
ip_address_column = None // The index of "IP address" column in the file
has_header_row = False // If the first row from the submitted file is a header row
remove_duplicate = True // If you want the system to remove duplicate emails
try:
response = zero_bounce.send_file(
file_path,
email_address_column,
return_url,
first_name_column,
last_name_column,
gender_column,
ip_address_column,
has_header_row,
remove_duplicate,
)
print("ZeroBounce send_file response: " + response)
except ZBException as e:
print("ZeroBounce send_file error: " + str(e))
SubmitParam.EmailAddressColumn := 1;
SubmitParam.IpAddressColumn := 2;
SubmitParam.HasHeaderRow := FALSE;
// submit csv file for bulk validation
FileFeedback := ZbBulkValidationFileSubmit(CSV_FILE_CONTENT, SubmitParam);
FileId := FileFeedback.FileId;
SubmitParam.EmailAddressColumn := 1;
SubmitParam.IpAddressColumn := 2;
SubmitParam.HasHeaderRow := FALSE;
// submit csv file for bulk validation
FileFeedback := ZbBulkValidationFileSubmit(CSV_FILE_CONTENT, SubmitParam);
FileId := FileFeedback.FileId;
File myFile = new File("<FILE_PATH>"); // The csv or txt file
int emailAddressColumn = 3; // The column index of the email address in the file. Index starts at 1
ZeroBounceSDK.getInstance().sendFile(
myFile,
emailAddressColumn,
null,
new ZeroBounceSDK.OnSuccessCallback<ZBSendFileResponse>() {
@Override
public void onSuccess(ZBSendFileResponse response) {
System.out.println("sendFile response=" + response.toString());
}
}, new ZeroBounceSDK.OnErrorCallback() {
@Override
public void onError(String errorMessage) {
System.out.println("sendFile error=" + errorMessage);
}
});
const payload = {
file: "<FILE>",
email_address_column: "<NUMBER_OF_COLUMN>", //example 3
return_url: "<RETURN_URL>", // (Optional)
first_name_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
last_name_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
gender_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
ip_address_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
has_header_row: true / false, // (Optional)
remove_duplicate: true / false, // (Optional)
};
try {
const response = await zeroBounce.sendFile(payload);
} catch (error) {
console.error(error);
}
const payload = {
file: "<FILE>",
email_address_column: "<NUMBER_OF_COLUMN>", //example 3
return_url: "<RETURN_URL>", // (Optional)
first_name_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
last_name_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
gender_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
ip_address_column: "<NUMBER_OF_COLUMN>", //example 3 (Optional)
has_header_row: true / false, // (Optional)
remove_duplicate: true / false, // (Optional)
};
try {
const response = await zeroBounce.sendFile(payload);
} catch (error) {
console.error(error);
}
var filePath = File("<FILE_PATH>"); // The csv or txt file
var options = new SendFileOptions();
options.ReturnUrl = "https://domain.com/called/after/processing/request";
options.EmailAddressColumn=3 // The index of "email" column in the file. Index starts at 1
options.FirstNameColumn = 4; // The index of "first name" column in the file
options.LastNameColumn = 5; // The index of "last name" column in the file
options.GenderColumn = 6; // The index of "gender" column in the file
options.IpAddressColumn = 7; // The index of "IP address" column in the file
options.HasHeaderRow = true; // If this is 'true' the first row is considered as table headers
ZeroBounce.Instance.SendFile(
filePath,
options,
response =>
{
Debug.WriteLine("SendFile success response " + response);
// ... your implementation
},
error =>
{
Debug.WriteLine("SendFile failure error " + error);
// ... your implementation
});
// import java.io.File
val myFile = File("<FILE_PATH>") // The csv or txt file
val emailAddressColumn = 3 // The column index of email address in the file. Index starts at 1
val firstNameColumn = 4 // The column index of first name in the file
val lastNameColumn = 5 // The column index of last name in the file
val genderColumn = 6 // The column index of gender in the file
val ipAddressColumn = 7 // The column index of IP address in the file
val hasHeaderRow = true // If this is 'true' the first row is considered as table headers
val returnUrl = "https://domain.com/called/after/processing/request"
ZeroBounceSDK.sendFile(
context,
file,
returnUrl,
firstNameColumn,
lastNameColumn,
genderColumn,
ipAddressColumn,
hasHeaderRow,
{ rsp ->
Log.d("MainActivity", "sendFile rsp: $rsp")
// your implementation
},
{ error ->
Log.e("MainActivity", "sendFile error: $error")
// your implementation
},
)
file, error_ := os.Open("PATH_TO_CSV_TO_IMPORT")
// ...check for error
defer file.Close()
// state either a positive number to state a column or exclude
// omit the column, if it does not exist in imported csv
csv_file := zerobouncego.CsvFile{
File: file,
HasHeaderRow: false,
EmailAddressColumn: 1,
FirstNameColumn: 2,
LastNameColumn: 3,
GenderColumn: 4,
IpAddressColumn: 5,
FileName: "emails.csv",
}
submit_response, error_ := zerobouncego.BulkValidationSubmit(csv_file, false)
// ...check for error
fmt.Println("submitted file ID: ", submit_response.FileId)
// #include <ZeroBounce/ZeroBounce.h>
ZeroBounce* zb = zero_bounce_get_instance();
zero_bounce_initialize(zb, "<YOUR_API_KEY>");
void on_error(ZBErrorResponse error_response) {
printf("%s
", zb_error_response_to_string(&error_response));
}
void on_success_send_file(ZBSendFileResponse response) {
printf("%s
", zb_send_file_response_to_string(&response));
}
char* file_path = "<FILE_PATH>";
int email_address_column = 3;
SendFileOptions options = new_send_file_options();
options.returnUrl = "https://domain.com/called/after/processing/request";
options.firstNameColumn = 4;
options.lastNameColumn = 5;
options.genderColumn = 6;
options.ipAddressColumn = 7;
options.hasHeaderRow = true;
options.removeDuplicate = true;
// #include <ZeroBounce/ZeroBounce.h>
ZeroBounce::getInstance()->initialize("<YOUR_API_KEY>");
std::string filePath = "<FILE_PATH>";
int emailAddressColumn = 3;
SendFileOptions options;
options.returnUrl = "https://domain.com/called/after/processing/request";
options.firstNameColumn = 4;
options.lastNameColumn = 5;
options.genderColumn = 6;
options.ipAddressColumn = 7;
options.hasHeaderRow = true;
options.removeDuplicate = true;
ZeroBounce::getInstance()->sendFile(
filePath,
emailAddressColumn,
options,
[](ZBSendFileResponse response) {
cout << response.toString() << endl;
},
[](ZBErrorResponse errorResponse) {
cout << errorResponse.toString() << endl;
}
);
ZeroBounce email API service features
Batch/Bulk email verification
The batch API endpoint allows you to batch up to 200 emails for cleaning simultaneously, with the ability to run up to 5 uses per minute. Get results in up to 70 seconds for each email address.
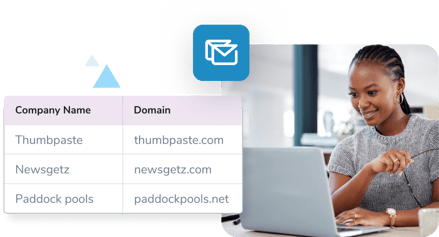
The world’s most accurate email verifier API

Valid emails
Genuine emails
Aliases
Alternate emails
Accept-all/Catch-all email
Easily spot catch-all emailsⓘAn email set up by a business to capture email messages sent to their name via an invalid address (e.g., j0hn@company.com instead of john@company.com). Catch-all inboxes receive a high volume of spam and may not be actively monitored by the owner. to further validate with AI Scoring
Do not mail
Global suppression
Traps
Toxic
Mx forward
Role-based catch-all

Invalid emails
Typos
Bad syntax
No DNS entries
Unroutable IP
Does not accept mail
Spam traps
Pristine traps
Typo traps
Recycled traps
Toxic domain verifier
Pinpoint domains that are popular for abuse and spam

Disposable emails
Detects temporary emails from a wide variety of disposable email providers
Abuse emails
Spot known complainers and remove them to keep spam complaint rate low
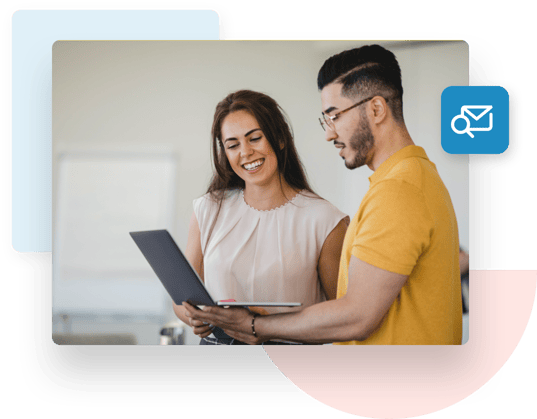
Getting started with the email verifier API
Using our email address checker API is easy. Here’s how to get started:
- Create your ZeroBounce account today - Free users get 100 free monthly email verification credits and 10 Email FinderⓘA tool that allows users to look up an email address by using either a name, an email domain, or a combination of both data points. queries.
- Get your API key - From your user-friendly dashboard, head over to API and grab your API key. You can generate up to 5 API keys.
- Connect your apps - Follow our user-friendly how-to docs to set up your preferred endpoints using languages like Javascript, PHP, Kottlin, and more.
PAY AS YOU GO!
2,000 credits
starting at $20.00
Email validation API pricing
At ZeroBounce, we keep pricing simple.
Cleaning emails with the email address checker API costs 1 ZeroBounce credit per email address. Use credits for Activity Data and AI Scoring at the cost of 1 additional credit per email per service.
You can also automatically top off your monthly credit balance and Email Finder queries with one of our convenient subscription plans.
Learn more about API pricing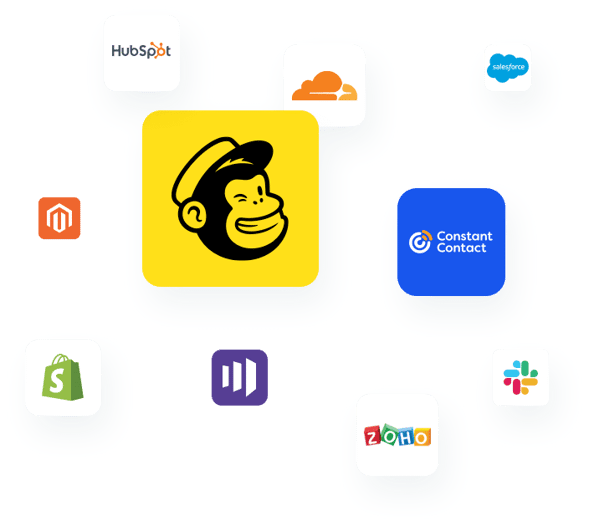
Seamless API integration with popular platforms
ZeroBounce’s list of native integrations now includes more than 50 global platforms. Take advantage of our email validation and Email Finder API with ease by adding your API key to CRMs and marketing platforms like HubSpot, Salesforce, Shopify, and more!
Check out our integrationsGet started with our email API service
There’s no faster way to zero bounces
Get 100 free email verifications
Discover more ZeroBounce API services
Frequently asked questions about our email API
You can manually or automatically validate email addresses individually or in bulk by installing the ZeroBounce email verifier API.
Once you create an account and obtain your API key, you can add our API to your favorite apps, platforms, websites, or landing pages via one of our supported languages. Then, call our API, and our email validator will validate your email addresses.
Learn more about how to install the API with our documentation.
Email verification API (Application Programming Interface) is a tool that allows you to use a service’s email verifier externally on your favorite apps and platforms. Integrating the API will enable you to upload your email list for verification or automatically check emails entered into your website registration forms.
An email API is a technology that allows other developers to submit and retrieve data and services from an external provider. Adding the email API to your preferred app or platform lets you enjoy the service’s benefits without leaving your preferred workspace.
No. The ZeroBounce email validation API uses the same verification credits you would use through our dashboard. API users will need a ZeroBounce account to obtain an API key and purchase credits for validation.
The batch email validator endpoint allows you to send up to 200 emails with a rate limit of 5 uses per minute. The single email validator and bulk file management endpoints feature no rate limit on the number of emails, files, or file sizes you may send.
The email verification API supports PHP for all endpoints. ZeroBounce users can leverage PHP for their bulk email verifier script, along with 16 total languages.
The email verification API currently supports the following languages:
• PHP
• iOS
• Scala
• Rust
• Ruby
• Python
• Pascal
• Delphi
• Java
• Javascript
• Node
• C#
• Android(Kotlin)
• Go
• C
• C++